Vim for Python Development
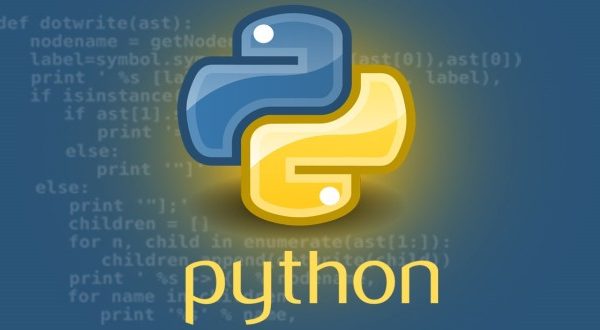
What is Vim?
Vim is a powerful text editor that belongs to one of the default components on every Linux distribution, as well as Mac OSX. Vim follows its own concept of usage, causing the community to divide into strong supporters and vehement opponents that are in favor for other editors like Emacs. (By the way, that’s very nice in winter in order to see the two enthusiastic teams having an extensive snowball fight together).
Vim can be individualized and extended using additional plugins in order to adjust the tool to your specific needs. In this article we highlight a selection of extensions and discuss a useful setup to improve software development with Python.
Auto-Completion
Vim is already equipped with an auto-completion feature. This works well but is limited to words that already exist in the current text buffer. In insert mode, using the key combination CTRL+N
you get the next word in the current buffer, and CTRL+P
the last one. In either way a menu with words pops up from which you choose the word to be pasted in the text at the current cursor position of the document.
This is already quite cool. Luckily, the same feature exists for entire lines of text. In insert mode press CTRL+X
first, followed by CTRL+L
. A menu pops up with similar lines from which you choose the line you would like to be pasted in the text at the current cursor position of the document.
To develop effectively in Python, Vim contains a standard module named pythoncomplete (Python Omni Completion). In order to activate this plugin add the following two lines to your Vim configuration file .vimrc
:
filetype plugin on
set omnifunc=syntaxcomplete#Complete
Then, in the Vim editor window the completion works in insert mode based on the key combination CTRL+X
followed by CTRL+O
. A submenu pops up that offers you Python functions and keywords to be used. The menu entries are based on Python module descriptions (“docstrings”). The example below shows the abs()
function with additional help on top of the vim editor screen.
The next plugin I’d like to discuss is named Jedi-Vim. It connects Vim with the Jedi autocompletion library.
Having installed the according package on your Debian GNU/Linux system it needs an additional step to make Jedi-Vim work. The plugin has to be activated using the Vim plugin manager as follows:
$ vim-addons install python-jedi
Info: installing removed addon 'python-jedi' to /home/frank/.vim
Info: Rebuilding tags since documentation has been modified ...
Processing /home/frank/.vim/doc/
Info: done.
Next, check the status of the plugin:
$ vim-addons status python-jedi
# Name User Status System Status
python-jedi installed removed
Now the plugin is activated and you can use it in Vim while programming. As soon as you either type a dot or press CTRL+Space
the menu opens and shows you method and operator names that could fit. The image below shows the according entries from the csv
module. As soon as you choose an item from the menu it will be pasted into your source code.
An interactive plugin is youcompleteme. It describes itself as “a fast, as-you-type, fuzzy-search code completion engine for Vim”. For Python 2 and 3, the automatic completion is based on Jedi as well. Among other programming languages it also supports C#, Go, Rust, and Java.
Provided in a Git repository, the setup requires additional steps in order to use it. The package on Debian GNU/Linux comes with a compiled version, and after installing the package via apt-get
the following steps will make it work. First, enable the package using the Vim Addon Manager (vam
) or the command vim-addons
:
$ vim-addons install youcompleteme
Info: installing removed addon 'youcompleteme' to /home/frank/.vim
Info: Rebuilding tags since documentation has been modified ...
Processing /home/frank/.vim/doc/
Info: done.
Next, check the status of the plugin. The output below shows you that the plugin is successfully installed for you as a regular user:
$ vim-addons status youcompleteme
# Name User Status System Status
youcompleteme installed removed
Third, copy the default ycm_extra_conf.py
file from the examples directory to your ~/.vim/
folder as follows:
$ cp -v /usr/share/doc/vim-youcompleteme/examples/ycm_extra_conf.py .ycm_extra_conf.py
"/usr/share/doc/vim-youcompleteme/examples/ycm_extra_conf.py" -> ".ycm_extra_conf.py"
The final step is to add the following two lines to your .vimrc
file:
" youcompleteme
let g:ycm_global_ycm_extra_conf = "~/.vim/.ycm_extra_conf.py"
The first line is a comment that could be omitted, and the second line defines the configuration file for the youcompleteme plugin. Et voila – now Vim accepts automated completion of code. When you see a useful completion string being offered, press the TAB
key to accept it. This inserts the completion string at the current position. Repeated presses of the TAB
key cycle through the offered completions.
Syntax Highlighting
Vim already comes with syntax highlighting for a huge number of programming languages that includes Python. There are three plugins that help to improve it – one is called python-syntax, the other one is python-mode, and the third one is python.vim.
Among others the python-syntax project site lists a high number of improvements such as highlighting for exceptions, doctests, errors, and constants. A nice feature is the switch between syntax highlighting for Python 2 and 3 based on an additional Vim command – :Python2Syntax
and :Python3Syntax
. This helps to identify possible changes that are required to run your script with both versions.
Combining Vim with the Revision Control System Git
Revision control is quite essential for developers, and Git is probably the best system for that. Compiling Python code, the interpreter creates a number of temporary files like __pycache__
and *.pyc
. The changes of these files need not to be tracked in Git. To ignore them Git offers the feature of a so-called .gitignore
file. Create this file in your Git-managed development branch with the following contents:
*.pyc
__pycache__
Also, add a README
file for your project to document what it is about. No matter how small your project is the README
file helps you (and others) to remember what the code is meant to do. Writing this file in Markdown format is especially helpful if you synchronize your Python code with your repository on GitHub. The README
file is rendered automatically to HTML that can be viewed easily in your web browser, then.
Vim can collaborate with Git directly using special plugins. Among others there is vim-fugitive, gv.vim and vimagit. All of them are available from Github, and mostly as a package for Debian GNU/Linux.
Having downloaded vim-fugitive via apt-get
it needs to be activated in a similar way as done before with the other plugins:
$ vim-addons install fugitive
Info: installing removed addon 'fugitive' to /home/frank/.vim
Info: Rebuilding tags since documentation has been modified ...
Processing /home/frank/.vim/doc/
Info: done
This plugin works with files that are tracked with Git, only. A large number of additional Vim commands becomes available such as :Gedit
, :Gdiff
, :Gstatus
, :Ggrep
and :Glog
. As stated on the project website these Vim commands correspond with the following Git commands and actions:
:Gedit
: Edit a file in the index and write to it to stage the changes:Gread
(git checkout -- filename
): Load the content of the file in the current buffer:Gwrite
(git add
): Add the file to the list of currently tracked files:Gdiff
(git diff
): Bring up the staged version of the file side by side with the working tree version and use Vim’s diff handling capabilities to stage a subset of the file’s changes:Gmove
(git mv
): Move a file to a new location:Gstatus
(git status
): Show the current status of your Git directory:Gcommit
(git commit
): Commit your changes:Ggrep
(git grep
): Search for the given pattern in the Git repository:Glog
(git log
): Loads all previous revisions of a file into the quickfix list so you can iterate over them and watch the file evolve!:Gblame
(git blame
): Shows who did the last changes to a file
Working With Skeletons
Skeleton files (or templates) are a nice feature of Vim that helps improve your productivity by adding default text to a file when a new one is created. For example, in many Python files you’ll have the a shebang, license, docstring, and author info at the beginning of the file. It would be a hassle to have to type or even copy this info to each file. Instead, you can use skeleton files to add this default text for you.
Let’s say, for example, you want all new Python files to start with the following text:
#!/user/bin/env python3
"""
[Add module documentation here]
Author: Frank
Date: [Add date here]
"""
You would create a file with this content and call it something like “skeleton.py”, and then move it to the directory ~/.vim/skeleton.py
. To tell Vim which file should be used as the skeleton file for Python, add the following to your .vimrc file:
au BufNewFile *.py 0r ~/.vim/skeleton.py
This tells Vim to use the specified skeleton file for all new files matching the filename “*.py”.
Notes on Using Plug-ins
Usually, Vim is quite fast. The more plugins you activate the longer it takes. The start of Vim is delayed, and takes noticeably longer than before. Also, it is common that Debian/Ubuntu packages work out of the box, and the installation scripts include all the steps to setup the plugin properly. I noticed that this is not the case, and sometimes additional steps are required.
More Resources
There are a number of courses and blog posts that cover various Vim settings for day-to-day use as a Python developer, which I’d highly recommend looking in to.
The following course aims for you to master Vim on any operating system, helping you gain a level of knowledge and comfortability with the editor that’s difficult to achieve by reading articles alone:
The rest are some great resources from around the web that we’ve found to be very helpful as well:
These articles help to extend your knowledge. Enjoy 🙂
Acknowledgements
The author would like to thank Zoleka Hatitongwe for her help and critical comments while preparing the article.