Variable-Length Arguments in Python with *args and **kwargs
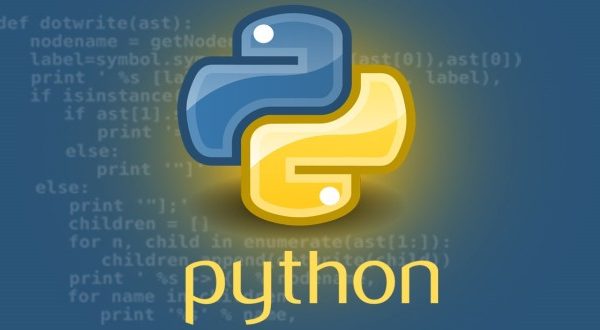
Introduction
Some functions have no arguments, others have multiple. There are times we have functions with arguments we don’t know about beforehand. We may have a variable number of arguments because we want to offer a flexible API to other developers or we don’t know the input size. With Python, we can create functions to accept any amount of arguments.
In this article, we will look at how we can define and use functions with variable length arguments. These functions can accept an unknown amount of input, either as consecutive entries or named arguments.
Using Many Arguments with *args
Let’s implement a function that finds the minimum value between two numbers. It would look like this:
def my_min(num1, num2):
if num1 < num2:
return num1
return num2
my_min(23, 50)
23
It simply checks if the first number is smaller than the second number. If it is, then the first number is returned. Otherwise, the second number is returned.
If we would like to find a minimum of 3 numbers, we can add another argument to my_min()
and more if-statements. If our minimum function needs to find the lowest number of any indeterminate amount,