The Python zip() Function
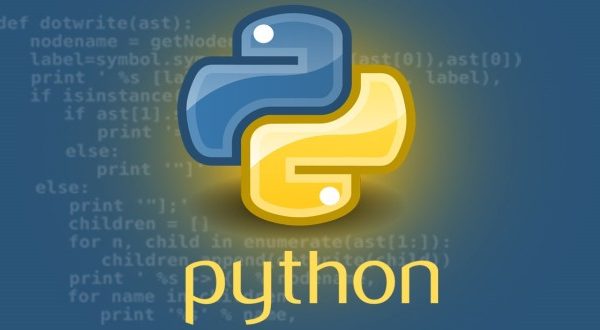
In this article, we’ll examine how to use the built-in Python zip()
function.
The zip()
function is a Python built-in function that allows us to combine corresponding elements from multiple sequences into a single list of tuples. The sequences are the arguments accepted by the zip()
function. Any number of sequences can be supplied, but the most common use-case is to combine corresponding elements in two sequences.
For example, let’s say we have the two lists below:
>>> vehicles = ['unicycle', 'motorcycle', 'plane', 'car', 'truck']
>>> wheels = [1, 2, 3, 4, 18]
We can use the zip()
function to associate elements from these two lists based on their order:
>>> list(zip(vehicles, wheels))
[('unicycle', 1), ('motorcycle', 2), ('plane', 3), ('car', 4), ('truck', 18)]
Notice how the output is a sequence of tuples, where each tuple combines elements of the input sequences with corresponding indexes.
One important thing to note is that if the input sequences are of differing lengths, zip()
will only match elements until the end of the shortest list is reached. For example:
>>> vehicles = ['unicycle', 'motorcycle', 'plane', 'car', 'truck']
>>> wheels = [1, 2, 3]
>>> list(zip(vehicles, wheels))
[('unicycle', 1),