Python: How to Remove a Key from a Dictionary
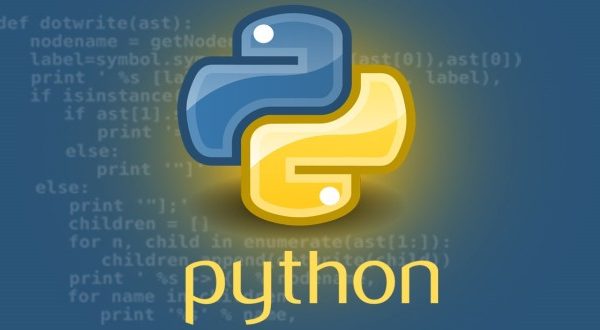
Introduction
In this article, we’ll take a look at how to remove keys from Python dictionaries. This can be done with the pop()
function, the del
keyword, and with dict comprehensions.
Remove a Key Using pop(key,d)
The pop(key, d)
function removes a key from a dictionary and returns its value. It takes two arguments, the key is removed and the optional value to return if the key isn’t found. Here’s an example of popping an element with only the required key
argument:
my_dict = {1: "a", 2: "b"}
popped_value = my_dict.pop(1)
line = "The value removed from the dictionary of the key: 1 is {}"
print(line.format(popped_value))
This snippet returns the following output:
The value removed from the dictionary of the key: 1 is a
Now, observe what happens when we try to remove a key that doesn’t exist:
my_dict = {1: "a",