Python: Check if Variable is a Number
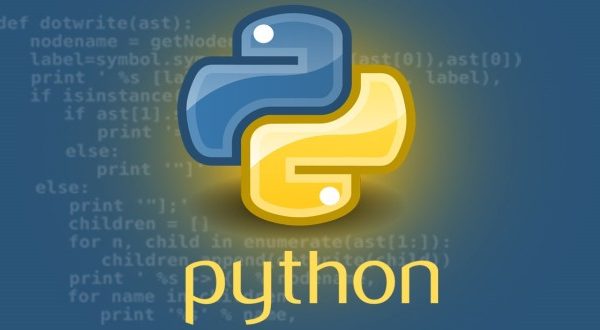
Introduction
In this article, we’ll be going through a few examples of how to check if a variable is a number in Python.
Python is dynamically typed. There is no need to declare a variable type, while instantiating it – the interpreter infers the type at runtime:
variable = 4
another_variable = 'hello'
Additionally, a variable can be reassigned to a new type at any given time:
# Assign a numeric value
variable = 4
# Reassign a string value
variable = 'four'
This approach, while having advantages, also introduces us to a few issues. Namely, when we receive a variable, we typically don’t know of which type it is. If we’re expecting a Number, but receive variable
, we’ll want to check if it’s a number before working with it.
Using the type() Function
The type()
function in Python returns the type of