Overview of Async IO in Python 3.7
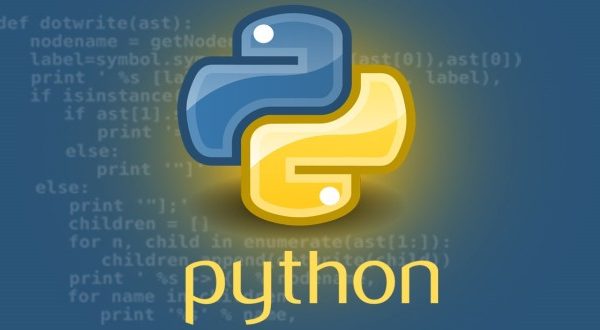
Python 3’s asyncio
module provides fundamental tools for implementing asynchronous I/O in Python. It was introduced in Python 3.4, and with each subsequent minor release, the module has evolved significantly.
This tutorial contains a general overview of the asynchronous paradigm, and how it’s implemented in Python 3.7.
Blocking vs Non-Blocking I/O
The problem that asynchrony seeks to resolve is blocking I/O.
By default, when your program accesses data from an I/O source, it waits for that operation to complete before continuing to execute the program.
with open('myfile.txt', 'r') as file:
data = file.read()
# Until the data is read into memory, the program waits here
print(data)
The program is blocked from continuing its flow of execution while a physical device is accessed, and data is transferred.
Network operations are another common source of blocking:
# pip install --user requests
import requests
req = requests.get('https://www.stackabuse.com/')
#
# Blocking occurs here, waiting for completion of an HTTPS request
#
print(req.text)
In many cases, the delay caused by blocking is negligible. However, blocking I/O scales very poorly. If you need to wait for 1010 file reads or network transactions, performance will suffer.