Introduction to Python Decorators
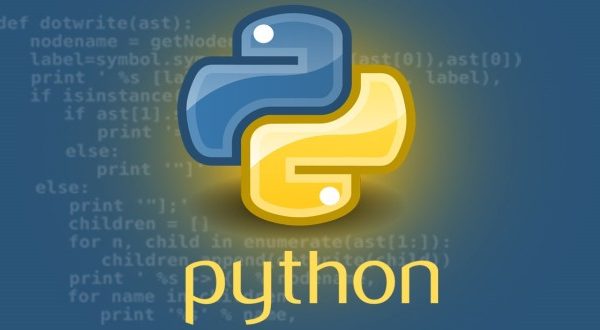
Introduction
In Python, a decorator is a design pattern that we can use to add new functionality to an already existing object without the need to modify its structure. A decorator should be called directly before the function that is to be extended. With decorators, you can modify the functionality of a method, a function, or a class dynamically without directly using subclasses. This is a good idea when you want to extend the functionality of a function that you don’t want to directly modify. Decorator patterns can be implemented everywhere, but Python provides more expressive syntax and features for that.
In this article, we will be discussing Python decorators in details.
How to Create Decorators
Let’s see how decorators can be created in Python. As an example, we will create a decorator that we can use to convert a function’s output string into lowercase. To do so, we need to create a decorator function and we need to define a wrapper inside it. Look at the following script:
def lowercase(func):
def wrapper():
func_ret = func()
change_to_lowercase = func_ret.lower()
return change_to_lowercase
return wrapper
In the script above, we have simply created a decorator named lowercase