How to Iterate over Rows in a Pandas DataFrame
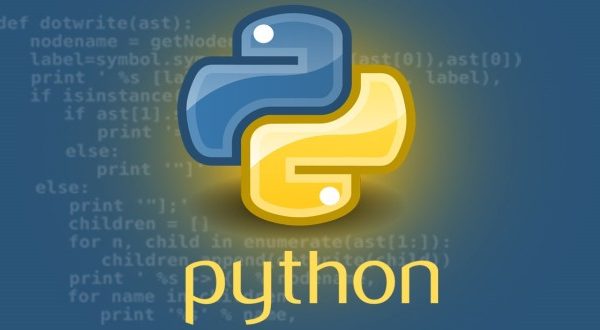
Introduction
Pandas is an immensely popular data manipulation framework for Python. In a lot of cases, you might want to iterate over data – either to print it out, or perform some operations on it.
In this tutorial, we’ll take a look at how to iterate over rows in a Pandas DataFrame
.
If you’re new to Pandas, you can read our beginner’s tutorial. Once you’re familiar, let’s look at the three main ways to iterate over DataFrame:
items()
iterrows()
itertuples()
Iterating DataFrames with items()
Let’s set up a DataFrame
with some data of fictional people:
import pandas as pd
df = pd.DataFrame({
'first_name': ['John', 'Jane', 'Marry', 'Victoria', 'Gabriel', 'Layla'],
'last_name': ['Smith', 'Doe', 'Jackson', 'Smith', 'Brown', 'Martinez'],
'age': [34, 29, 37, 52, 26, 32]},
index=['id001', 'id002', 'id003', 'id004', 'id005', 'id006'])
Note that we are using id’s as our DataFrame
‘s index. Let’s take a look at