Getting User Input in Python
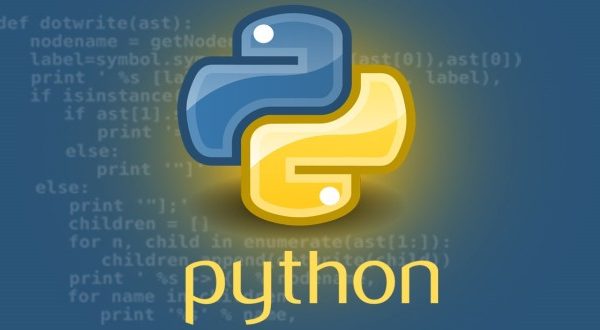
Introduction
The way in which information is obtained and handled is one of the most important aspects in the ethos of any programming language, more so for the information supplied and obtained from the user.
Python, while comparatively slow in this regard when compared to other programming languages like C or Java, contains robust tools to obtain, analyze, and process data obtained directly from the end user.
This article briefly explains how different Python functions can be used to obtain information from the user through the keyboard, with the help of some code snippets to serve as examples.
Input in Python
To receive information through the keyboard, Python uses either the input()
or raw_input()
functions (more about the difference between the two in the following section). These functions have an optional parameter, commonly known as prompt
, which is a string that will be printed on the screen whenever the function is called.
When one of the input()
or raw_input()
functions is called, the program flow stops until the user enters the input via the command line. To actually enter the data, the user needs to press the ENTER key after inputing their string. While hitting the ENTER key usually inserts a newline character (“n”), it does not in this case. The entered string will simply be submitted to the application.
On a curious note, little has changed in how this function works between Python versions 2 and 3, which is reflected in the workings of input()
and raw_input()
, explained in the next section.
Comparing the input and raw_input Functions
The difference when using these functions only depends on what version of Python is being used. For Python 2, the function raw_input()
is used to get string input from the user via the command line, while the input()
function returns will actually evaluate the input string and try to run it as Python code.
In Python 3, raw_input()
function has been deprecated and replaced by the input()
function and is used to obtain a user’s string through the keyboard. And the input()
function of Python 2 is discontinued in version 3. To obtain the same functionality that was provided by Python 2’s input()
function, the statement eval(input())
must be used in Python 3.
Take a look at an example of raw_input
function in Python 2.
# Python 2
txt = raw_input("Type something to test this out: ")
print "Is this what you just said?", txt
Output
Type something to test this out: Let the Code be with you!
Is this what you just said? Let the Code be with you!
Similarly, take a look at an example of input function in Python 3.
# Python 3
txt = input("Type something to test this out: ")
# Note that in version 3, the print() function
# requires the use of parenthesis.
print("Is this what you just said? ", txt)
Output
Type something to test this out: Let the Code be with you!
Is this what you just said? Let the Code be with you!
From here onwards this article will use the input
method from Python 3, unless specified otherwise.
String and Numeric input
The input()
function, by default, will convert all the information it receives into a string. The previous example we showed demonstrates this behavior.
Numbers, on the other hand, need to be explicitly handled as such since they come in as strings originally. The following example demonstrates how numeric type information is received:
# An input is requested and stored in a variable
test_text = input ("Enter a number: ")
# Converts the string into a integer. If you need
# to convert the user input into decimal format,
# the float() function is used instead of int()
test_number = int(test_text)
# Prints in the console the variable as requested
print ("The number you entered is: ", test_number)
Output
Enter a number: 13
The number you entered is: 13
Another way to do the same thing is as follows:
test_number = int(input("Enter a number: "))
Here we directly save the input, after immediate conversion, into a variable.
Keep in mind that if the user doesn’t actually enter an integer then this code will throw an exception, even if the entered string is a floating point number.
Input Exception Handling
There are several ways to ensure that the user enters valid information. One of the ways is to handle all the possible errors that may occur while user enters the data.
In this section we’ll demonstrates some good methods of error handling when taking input.
But first, here is some unsafe code:
test2word = input("Tell me your age: ")
test2num = int(test2word)
print("Wow! Your age is ", test2num)
When running this code, let’s say you enter the following:
Tell me your age: Three
Here, when the int()
function is called with the “Three” string, a ValueError exception is thrown and the program will stop and/or crash.
Now let’s see how we would make this code safer to handle user input:
test3word = input("Tell me your lucky number: ")
try:
test3num = int(test3word)
print("This is a valid number! Your lucky number is: ", test3num)
except ValueError:
print("This is not a valid number. It isn't a number at all! This is a string, go and try again. Better luck next time!")
This code block will evaluate the new input. If the input is an integer represented as a string then the int()
function will convert it into a proper integer. If not, an exception will be raised, but instead of crashing the application it will be caught and the second print
statement is run.
Here is an example of this code running when an exception is raised:
Tell me your lucky number: Seven
This is not a valid number. It isn't a number at all! This is a string, go and try again. Better luck next time!
This is how input-related errors can be handled in Python. You can combine this code with another construct, like a while loop to ensure that the code is repeatedly run until you receive the valid integer input that your program requires.
A Complete Example
# Makes a function that will contain the
# desired program.
def example():
# Calls for an infinite loop that keeps executing
# until an exception occurs
while True:
test4word = input("What's your name? ")
try:
test4num = int(input("From 1 to 7, how many hours do you play in your mobile?" ))
# If something else that is not the string
# version of a number is introduced, the
# ValueError exception will be called.
except ValueError:
# The cycle will go on until validation
print("Error! This is not a number. Try again.")
# When successfully converted to an integer,
# the loop will end.
else:
print("Impressive, ", test4word, "! You spent", test4num*60, "minutes or", test4num*60*60, "seconds in your mobile!")
break
# The function is called
example()
The output will be:
What's your name? Francis
From 1 to 7, how many hours do you play in your mobile? 3
Impressive, Francis! You spent 180 minutes or 10800 seconds on your mobile!
Conclusion
In this article, we saw how the built-in Python utilities can be used to get user input in a variety of formats. We also saw how we can handle the exceptions and errors that can possibly occur while obtaining user input.