Daily Coding Problem: Programming Puzzles to your Inbox
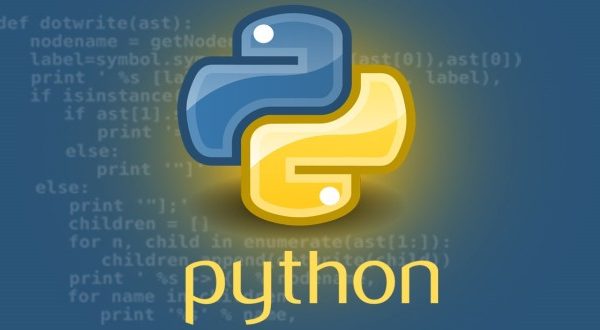
Like just about any other profession, the key to becoming a great programmer is to practice. Practicing often and consistently is an amazing way, and arguably the best way, to challenge yourself and improve your programming skills.
A lot of us have the desire to work in top-tier tech companies, like Microsoft, Google, Facebook, etc. Although a lot of people are scared to even attempt to apply to such high-caliber jobs, feeling too intimidated or under-qualified for a position at one of those companies.
Going to an interview with a top company, or any interview, clear-headed and prepared is important, as looking confident and competent in an interview can mean the difference between landing your dream job or being rejected.
There’s just so many things a person can do to become confident for such an interview, and in my opinion – the absolute best way is simply to practice. Being able to solve a problem on the spot tells the interviewer more about your skills than anything you can say verbally.
There are many ways a person can practice, whether it be on personal problems and projects, other resources like books, or using other services online. Daily Coding Problem is one such service.
What is Daily Coding Problem?
Daily Coding Problem is a simple and very useful platform that emails you one coding problem to solve every morning. This ensures that you practice consistently and often enough to stay in shape over a long period of time.
Practicing just one problem a day is enough to make a huge impact on your skill-set and confidence, especially when faced with a task that you might be facing in a top-tier tech company in the near future.
The best thing about their problems is that they use actual problems and questions from top companies that other candidates have faced in their interview sessions there. And personally, I agree with their philosophy – it’s always better to be over-prepared rather than under-prepared.
Anyone can use their service, whether you’re a self-taught aspiring developer, a recent college graduate, or an experienced professional developer looking to sharpen your skills. What matters most is your understanding of the concepts, data structures, and algorithms you have at your disposal to solve a given problem.
And one of the best things about DCP is that you get these coding problems to your inbox for free. For a small fee, they’ll also send you the solutions and in-depth explanations for every single problem you get emailed to your inbox, which you’ll receive the day after the problem, giving you time to work it out on your own.
An Example Problem
With each problem emailed to you, you’ll get the company of origin, the formulation of the problem, and a code example. In this section we’ll take a look at one of DCP’s coding problems and the corresponding detailed solution:
Given two singly linked lists that intersect at some point, find the intersecting node. The lists are non-cyclical.
For example, given A = 3 -> 7 -> 8 -> 10 and B = 99 -> 1 -> 8 -> 10, return the node with value 8.
In this example, assume nodes with the same value are the exact same node objects.
Do this in O(M + N) time (where M and N are the lengths of the lists) and constant space.
This problem was asked by Google in an actual interview.
Take your time to carefully read this problem and think a bit about how you would solve it before proceeding. According to the DCP’s FAQ, you should have around an hour or so to solve these problems in the interviews, so that should also be the timeframe you allow yourself to solve them at home.
Once you think you’ve figured it out, or when you get stuck, you can take a look at the detailed solution provided by Daily Coding Problem:
We might start this problem by first ignoring the time and space constraints, in order to get a better grasp of the problem.
Naively, we could iterate through one of the lists and add each node to a set or dictionary, then we could iterate over the other list and check each node we're looking at to see if it's in the set. Then we'd return the first node that is present in the set. This takes O(M + N) time but also O(max(M, N)) space (since we don't know initially which list is longer). How can we reduce the amount of space we need?
We can get around the space constraint with the following trick: first, get the length of both lists. Find the difference between the two, and then keep two pointers at the head of each list. Move the pointer of the larger list up by the difference, and then move the pointers forward in conjunction and check if they match.
def length(head):
if not head:
return 0
return 1 + length(head.next)
def intersection(a, b):
m, n = length(a), length(b)
cur_a, cur_b = a, b
if m > n:
for _ in range(m - n):
cur_a = cur_a.next
else:
for _ in range(n - m):
cur_b = cur_b.next
while cur_a != cur_b:
cur_a = cur_a.next
cur_b = cur_b.next
return cur_a
DCP offers in-depth and detailed explanations like this to help you solve each problem and actually understand the solution, as well as reinforce the logic you utilized if you were able to solve it on your own.
As you can see, these problems rely heavily on both logic and creativity, which is what makes them very difficult to solve. But once you’ve practiced enough and learn the tricks behind these problems – like how to think about the problem and solution, and what tools you have at your disposal – they will become much easier.
Which Programming Languages?
Currently, DCP provides solutions in Python as it is very well-known and similar to pseudo-code, as well as being simple to learn. This seems to be a good choice for solutions since Python code is fairly easy to translate to other languages, given its simple syntax and straight-forward programming style.
Additionally, DCP is looking to expand their pool of solutions to other languages as well, like Java, JavaScript, C++, and Ruby.
Pricing
The best part about all of this is that it’s absolutely free to sign up for their service and receive problems to solve each morning.
To get the solutions emailed to you, on the other hand, requires that you pay a small fee:
The yearly plan charges you only $7.50/month (billed annually) for their services – that’s a tad more than a cup of Starbucks coffee in Germany, or around the price of two average cups of coffee in the US!
For the price of a couple of cups of coffee, each month you will receive about 30 real-life interview problems to solve and improve your skills and confidence vastly. It’s hard to find another investment that’ll pay off this well.
Other Resources for Learning
Lucky for you, Daily Coding Problem isn’t the only place to practice and learn online!
If books are more your thing, make sure to take a look at these:
If you’re preparing for a big interview, I’d also suggest that you read up on some tips that will help you improve your chances of landing the job:
Or if you’re interested in reading articles on some of the most in-demand and popular programming languages in the world today, check out our Node, Python, or Java articles.
Happy coding!