Course Review: Master the Python Interview
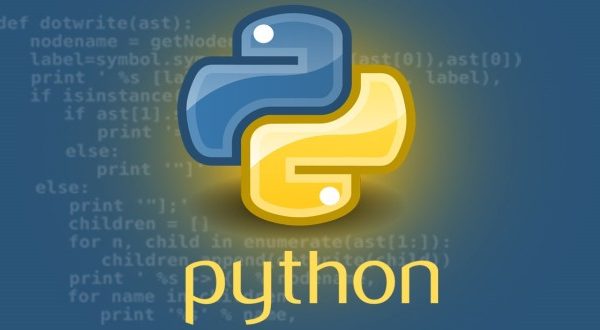
Introduction
This article will be a continuation of the topic of my prior article Preparing for a Python Developer Interview where I gave my opinions and suggestions that I feel will put you in the best position to out perform other developers competing for a Python developer role. In this article I will be reviewing the popular Udemy course on preparing for a Python developer interview by Nicolas Georges called Master the Python Interview – get the senior & well paid job.
Before getting started, we should mention that there are a lot of ways to practice programming interview questions, including services like Daily Coding Problem, which will email you a new problem to solve every day.
Structure and Topics Covered in the Course
The structure of this course is composed of sections covering the topics listed below where each section ends in either one or more exercises or quizzes to reinforce the material.
The topics covered by Nicolas in his course are as follows:
- Collections with Lists and Tuples
- Intro to OOP in Python
- Unit testing
- Idiomatic Python – Ask for forgiveness not permission
- Must know Python Programming Constructs
- Must know Python Data Structures
- More on OOP in Python
- Data Structure Comprehensions
In the sections that follow I briefly discuss the content of each section along with things that I liked and did not like about each. I conclude with an additional section discussing things that I feel would benefit this Udemy course if they were included or done differently.
Before I get into the individual sections I would like to note that this course was taught using “legacy” Python 2.7 which I feel is a bit of a flaw in the course. The Python community is just over a year away from completely loosing support from the Core developer team with regards to maintenance of Python 2. For this reason I feel it necessary for Python content producers to 100 percent adopt and use Python 3.
Collections with Lists and Tuples
Collections are an enormously important topic in all high level programming languages and Python is certainly no exception to this, so I am quite glad that they were covered in this course. Nicolas does a good job of differentiating between immutability and mutability in relation to lists and tuples which, in my opinion, are the primary differentiators between the two.
Unfortunately there was a charge made about implementation of lists and tuples that I found to be either very misleading or flat out incorrect. In this section Nicolas states that “lists contain homogenous data types while tuples are meant to contain heterogenous data types”. At first I thought this was simply a harmless gaffe that all of are susceptible to in life, but later in this section it was reiterated and it was even reenforced in one of the section ending quizzes.
I would like to take some time to correct this statement as I believe that Nicolas was probably trying to describe a common usage trend where lists often contain homogenous data types while tuples can often contain heterogenous data types. In my experience it is true that when I use lists the data in them are usually of the same type. However, it is important to know that both lists and tuples can in fact contain different data types as well as the same.
Here is an example of lists and tuples containing the same data types which are strings representing the letters of my name:
>>> x = ['a','d', 'a', 'm']
>>> y = ('a', 'd', 'a', 'm')
And here is an example of lists and tuples containing different data types of a string representing my name and an integer representing my age:
>>> x = ['Adam', 30]
>>> y = ('Adam', 30)
Intro to OOP in Python
In this section Nicolas explains a very important feature of the Python programming language in that every single element of the language is in the form of an object. From this you can extrapolate that the language is a fully object oriented language. Nicolas goes on to demonstrate and explain the usage and usefulness of many built-in functions that enable to the programmer to inspect objects like dir()
, id()
, help()
as well as others.
However, Nicolas does contradict his earlier statements about homogeny / heterogeneity of data types in lists during this section, which I hope can get cleaned up as I believe most early Python users would become quite confused at this point of the course.
Unit testing
I was most impressed with this section of the course. I feel many, if not most, of the courses on programming often fail to address the importance of testing one’s code. Nicolas does an excellent job covering the basics of the unittest
module and even devotes considerable time explaining how to use test driven development and why it is important.
Idiomatic Python – Ask for forgiveness not permission
This is the part of the course where Nicolas begins to transition into common conventions, or idioms, of the Python programming community. I do not want to steal Nicolas’s thunder by going too far into the explanation of the material covered here because I believe he does a great job explaining what it means to “ask for forgiveness and not permission” and how this convention differs in Python as opposed to other languages, such as Java.
Must know Python Programming Constructs
I was a little confused at why this section of the courses exists and why it was placed in the middle of the course. The topics covered in this section go over the very basic syntactic constructs like boolean expressions, conditionals, and loops. For a course targeting mid to senior level Python developers it felt like this section should be assumed knowledge, but I guess for completeness it is not inappropriate to include it. I do think it would perhaps make better sense to put this material at the beginning of the course, however.
With the above said about this section I do want to leave my review of this section with something that I did find quite positive. I liked that Nicolas explained what it meant in the language to be considered truthy / falsy, to steal a term from the Javascript community. Nicolas did a great job of taking the time to describe the usefulness of the built-in bool()
function to test for boolean equivalents to commonly used conditional expressions to test values such as empty lists, empty strings, None, and others.
Must know Python Data Structures
Here Nicolas introduces an additional collection data type, which is known as a set
and follows with a comparisons of sets and lists. During this explanation he covers the notion of what it means to be hashable.
However, one thing that I felt was missing here was an explanation of performance benefits of searching a set for inclusion of a value as compared to a list, which is a major benefit of using sets.
More on OOP in Python
This section circles back around to further elaborate on OOP in Python. Nicolas further explains the syntax and meaning of defining a custom class and creating objects from it. He introduces the concepts of defining custom instance attributes and methods as well as goes into what magic methods are and how they are used. In general I felt this section was well covered and is important knowledge for a mid-to-senior level Python developer.
Data Structure Comprehensions
The course finishes with a section on one of my favorite Pythonic features, comprehensions. Here Nicolas demonstrates how comprehensions are used and why you might use them when working with lists and dictionaries.
Topics to Add that would Benefit the Course
Given that the title of this course indicates that its target audience is geared towards mid-to-senior level Python developer roles I feel that not enough content was aimed at more describing more mid-level to advanced features of the language. Below are a set of topics that I believe would elevate the course to better suit its target audience.
A. More idiomatic Python programming techniques are in order. An example of what I mean by this is simply unpacking of tuples and lists into component elements. I see this often demonstrated in advanced texts as well as blogs and personally find it to be congruent with the well known Python idiom that explicit is better than implicit.
I think a coding example would better demonstrate my argument here. Consider the case where you have a list of tuples where each tuple represents the length and width of a rectangle and you would like to iterate over them to calculate and display each one’s area. I can think of two variations in which I might implement this: (i) one uses indexing of the tuple elements, and (ii) the other utilizes tuple unpacking into meaningfully named variables.
Using indexing:
>>> shapes = [(1,1), (2,2), (3,2)]
>>> for shape in shapes:
... print "Area of shape %.2f" % (shape[0] * shape[1])
...
Area of shape 1.00
Area of shape 4.00
Area of shape 6.00
Using unpacking:
>>> for width, height in shapes:
... print "Area of shape %.2f" % (width * height)
...
Area of shape 1.00
Area of shape 4.00
Area of shape 6.00
To me the second example that uses unpacking is more readable and demonstrates a greater idiomatic Python implementation.
B. A discussion of built-in Python functions that perform operations on collections would be a great addition to this course. Many of the built-in functions have been provided because they provide solutions to common programming problems, but have highly optimized implementations that often give significant performance boosts. Some of the built-in functions that I think would be worth mentioning are zip, filter, and map.
For example, say you want to filter a list of numbers and only select those that are even. I can think of two common approaches that would be taken. One that would use a loop to iterate over the items along with a conditional to test each number to see if it is even or not and when even add the number to a separate lists designated for the even numbers. This is likely to be the approach taken by a junior developer who is less familiar with the language. The second would be to use the built in filter()
function along with a lambda function to test for even numbers.
In code these two approaches would look like so:
First method:
>>> nums = [1, 2, 3, 4, 5, 6, 7, 8]
>>> even_nums = []
>>> for num in nums:
... if num % 2 == 0:
... even_nums.append(num)
...
>>> even_nums
[2, 4, 6, 8]
Second method:
>>> even_nums = filter(lambda num: num % 2 == 0, nums)
>>> even_nums
[2, 4, 6, 8]
C. Another topic that I think would be beneficial to add to the existing content would be coverage of some of the advanced collection data types such as named tuples and ordered dictionaries. I have often reached for a named tuple in cases where I wanted to represent a real world object but, would be maybe awkward or overkill to use a custom class or the overly used dictionary. Not only are they a great way to organize data representing something in the real word but they have excellent performance, particularly better than a dictionary.
D. Last but certainly not least I would really have liked to see mention of the differences between Python 2 and 3. In particular I feel it would have been important to give some pointers for migrating existing systems from Python 2 to Python 3 which is quickly becoming a priority item for many companies and leading to increased demand for senior Python developers.
Conclusion
In this article I have done my best to give a thorough and honest review of the Udemy course, Master the Python Interview – get the senior & well paid job by Nicolas Georges, which currently has seen about 2,700 enrollments.
My overall opinion of the course is that it is a bit misleading because it’s title leads one to believe that the content is geared more towards the mid-to-senior level Python developer, while I found it to be a bit lacking for that. That being said there is some really excellent content covered in this course that will be valuable to entry and junior level Python devs.
As always I thank you for reading and welcome comments and criticisms below.