Convenient script for trading with python
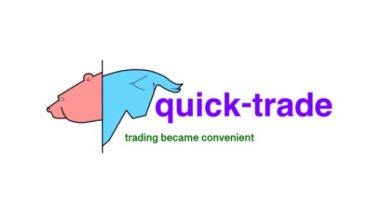
quick_trade
convenient script for trading with python.
used:
├──ta by Darío López Padial (Bukosabino https://github.com/bukosabino/ta)
├──tensorflow (https://github.com/tensorflow/tensorflow)
├──pykalman (https://github.com/pykalman/pykalman)
├──plotly (https://github.com/plotly/plotly.py)
├──scipy (https://github.com/scipy/scipy)
├──pandas (https://github.com/pandas-dev/pandas)
├──numpy (https://github.com/numpy/numpy)
└──iexfinance (https://github.com/addisonlynch/iexfinance)
Algo-trading system with python.
customize your strategy!
import quick_trade.trading_sys as qtr
from quick_trade import brokers
import yfinance as yf
import ccxt
class My_trader(qtr.Trader):
def strategy_sell_and_hold(self):
ret = []
for i in self.df['Close'].values:
ret.append(qtr.utils.SELL)
self.returns = ret
self.set_credit_leverages(1.0)
self.set_open_stop_and_take()
return ret
a = My_trader('MSFT', df=yf.download('MSFT', start='2019-01-01'))
a.set_pyplot()
a.set_client(brokers.TradingClient(ccxt.binance()))
a.strategy_sell_and_hold()
a.backtest()
find the best strategy!
import quick_trade.trading_sys as qtr
import ta.trend
import ta.momentum
import ta.volume
import ta.others
import ta.volatility
import ccxt
from quick_trade.quick_trade_tuner.tuner import *
class Test(qtr.Trader):
def strategy_bollinger_break(self, **kwargs):
self.strategy_bollinger(plot=True, **kwargs)
self.inverse_strategy(swap_tpop_take=False)
self.set_open_stop_and_take()
self.set_credit_leverages()
self.convert_signal()
return self.returns
def bb(self, **kwargs):
self.strategy_bollinger(plot=False, **kwargs)
self.set_open_stop_and_take()
self.set_credit_leverages()
self.convert_signal()
return self.returns