Bucket Sort in Python
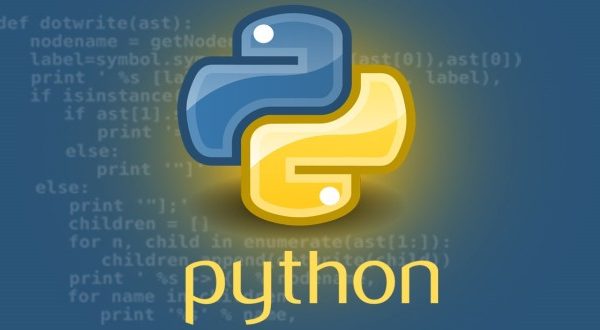
Introduction
In this tutorial, we’ll be diving into the theory and implementation of Bucket Sort in Python.
Bucket Sort is a comparison-type algorithm which assigns elements of a list we want to sort in Buckets, or Bins. The contents of these buckets are then sorted, typically with another algorithm. After sorting, the contents of the buckets are appended, forming a sorted collection.
Bucket Sort can be thought of as a scatter-order-gather approach towards sorting a list, due to the fact that the elements are first scattered in buckets, ordered within them, and finally gathered into a new, sorted list.
We’ll implement Bucket Sort in Python and analyze it’s time complexity.
How does Bucket Sort Work?
Before jumping into its exact implementation, let’s walk through the algorithm’s steps:
- Set up a list of empty buckets. A bucket is initialized for each element in the array.
- Iterate through the bucket list and insert elements from the array. Where each element is inserted depends on the input list and the largest element of it. We can end up with
0..n
elements in each bucket. This will be elaborated on in the visual presentation of the algorithm. - Sort each non-empty bucket.